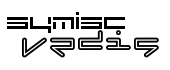
An Embeddable Datastore Engine |
Tweet |
Follow @Vedis |
Vedis in 5 Minutes or Less.
Here is what you do to start experimenting with Vedis without having to do a lot of tedious reading and configuration:
download The Code
Get a copy of the last public release of Vedis. Visit the download page for additional information.
Some C/C++ samples to start experimenting with:
- vedis_intro.c : Introduction to the command execution (i.e. GET, SET, HMGET, SLEN, etc.) API.
- vedis_kv_intro.c: Introduction to the Raw Key/Value store API.
- vedis_shell.c: Source code of the Vedis shell which let you execute built-in commands from your CLI.
Write Programs That Use Vedis
The principal task of a datastore engine is to store and retrieve records as fast as possible. Vedis support both structured and raw data storage.
Structured data storage is presented to clients via the command execution interface (CEI). Basically, you execute one or more commands ala Redis (i.e. SET key value; GET key, HSET...) via vedis_exec() and you extract the execution result (The return value of the command) via vedis_exec_result(). Refer to the following page for the list of built-in commands.
Raw data storage is presented to clients via the Key/Value store interfaces. Vedis is a standard key/value store similar to Berkeley DB, Tokyo Cabinet, LevelDB, etc. but with a rich feature set including support for transactions (ACID), concurrent reader, etc.
Under the KV store, both keys and values are treated as simple arrays of bytes, so content can be anything from ASCII strings, binary blob and even disk files. The KV store layer is presented to clients via a set of interfaces, these includes: vedis_kv_store(), vedis_kv_append(), vedis_kv_fetch_callback(), vedis_kv_append_fmt(), etc.
Introduction to the
command Execution interfaces (CEI)
Below
is a simple C program that demonstrates how to use the Command Execution C/C++
interface to Vedis.
- vedis *pStore; /* Datastore handle */
- int rc;
- /* Create our datastore */
- rc = vedis_open(&pStore,argc > 1 ? argv[1] /* On-disk DB */ : ":mem:"/* In-mem DB */);
- if( rc != VEDIS_OK ){ /* Seriously? */ return; }
-
- /* Execute the simplest command */
- rc = vedis_exec(pStore,"SET test 'Hello World'",-1);
- if( rc != VEDIS_OK ){ /* Handle error */ }
- /* Another simple command (Multiple set) */
- rc = vedis_exec(pStore,"MSET username james age 27 mail dude@example.com",-1);
- if( rc != VEDIS_OK ){ /* Handle error */ }
- /* A quite complex command (Multiple hash set) using foreign data */
- rc = vedis_exec_fmt(pStore,
- "HMSET config pid %d user %s os %s scm %s",
- 1024 /* pid */,
- "dean", /* user */
- "FreeBSD", /* OS */
- "Git" /* SCM */
- );
- if( rc != VEDIS_OK ){ /* Handle error */ }
- /* Fetch some data */
- rc = vedis_exec(pStore,"GET test",-1);
- if( rc != VEDIS_OK ){ /* Seriously? */ }
- /* Extract the return value of the last executed command (i.e. 'GET test') " */
- vedis_exec_result(pStore,&pResult);
- {
- const char *zResponse;
- /* Cast the vedis object to a string */
- zResponse = vedis_value_to_string(pResult,0);
- /* Output */
- printf(" test ==> %s\n",zResponse); /* test ==> 'Hello world' */
- }
- vedis_exec(pStore,"GET mail",-1);
- /* 'GET mail' return value */
- vedis_exec_result(pStore,&pResult);
- {
- const char *zResponse;
- /* Cast the vedis object to a string */
- zResponse = vedis_value_to_string(pResult,0);
- /* Output */
- printf(" mail ==> %s\n",zResponse); /* Should be 'dude@example.com' */
- }
- /*
- * A command which return multiple value in array.
- */
- vedis_exec(pStore,"MGET username age",-1); /* james 27*/
- vedis_exec_result(pStore,&pResult);
-
- if( vedis_value_is_array(pResult) ){
- /* Iterate over the elements of the returned array */
- vedis_value *pEntry;
- puts("Array entries:");
- while((pEntry = vedis_array_next_elem(pResult)) != 0 ){
- const char *zEntry;
- /* Cast to string and output */
- zEntry = vedis_value_to_string(pEntry,0);
- /* Output */
- printf("\t%s\n",zEntry);
- }
- }
-
- /* Extract hashtable data */
- vedis_exec(pStore,"HGET config pid",-1); /* 1024 */
- vedis_exec_result(pStore,&pResult);
- {
- int pid;
- /* Cast to integer */
- pid = vedis_value_to_int(pResult);
- /* Output */
- printf("pid ==> %d\n",pid); /* Should be 1024 */
- }
- /* Finally, auto-commit the transaction and close our datastore */
- vedis_close(pStore);
-
The datastore is created on line 5 using a call to vedis_open(). This is often the first Vedis API call that an application makes and is a prerequisite in order to play with Vedis.
-
As you can see, executing commands (ala Redis) under Vedis is pretty simple and involve only a single call to vedis_exec() or vedis_exec_fmt(). This is done on line 9, 13, 17, 45 on our example regardless how much the executed command is complex.
-
Execution result (i.e. Return value) of the last executed command is extracted via vedis_exec_result(). This is done on line 27, 31, 40, 54 in our example.
-
After that, the host application need to cast this object value (i.e. Return value of the last executed command) to the desired C type (String, Integer, Double or Boolean). For this purpose, a set of smart interfaces is available: vedis_value_to_string(), vedis_value_to_int(), vedis_value_to_bool(), etc. This done on line 35, 46, 64 and 76.
-
Working with commands which returns an object value of type array (i.e. MGET, HMGET, HVALS, CMD_LIST, etc.) is straightforward and involve only a single call to vedis_array_walk() or vedis_array_next_elem(). The first interface expect a user supplied callback which is invoked for each entry in the array while the latter iterate over the array elements one after one.
-
Finally, the transaction is auto-committed and our datastore is closed on line 81 using the vedis_close() interface.
Enhancing Vedis with foreign (i.e. User defined) commands is pretty simple and involve only a single call to vedis_register_command(). All of the built-in commands are installed using exactly this interface.
Introduction to the Key/Value Store Interfaces
By far, the Vedis Key/Value store layer is the simplest, yet the most flexible since it does not impose any structure or schema to the database records.
The Key/Value store layer is presented to clients via a set of interfaces, these includes: vedis_kv_store(), vedis_kv_append(), vedis_kv_fetch(), vedis_kv_append_fmt(), and many more.
Below is a simple C program that demonstrates how to use the Key/Value C/C++ interface to Vedis.
- vedis *pStore; /* Datastore handle */
- int rc;
- /* Create our datastore */
- rc = vedis_open(&pStore,argc > 1 ? argv[1] /* On-disk DB */ : ":mem:" /* In-mem DB */);
- if( rc != VEDIS_OK ){ /* Seriously? */ return; }
- /* Store some records */
- rc = vedis_kv_store(pStore,"test",-1,"Hello World",11); /* test => 'Hello World' */
- if( rc != VEDIS_OK ){ /* Handle error */ }
- /* A small formatted string */
- rc = vedis_kv_store_fmt(pStore,"date",-1,"dummy date: %d:%d:%d",2013,9,17); /* Dummy date */
- if( rc != VEDIS_OK ){ /* Handle error */ }
-
- /* Switch to the append interface */
- rc = vedis_kv_append(pStore,"msg",-1,"Hello, ",7); //msg => 'Hello, '
- if( rc != VEDIS_OK ){ /* Handle error */ }
- /* The second chunk */
- rc = vedis_kv_append(pStore,
- "msg",-1,"dummy time is: ",17 /* msg => 'Hello, Current time is: '*/
- );
- if( rc != VEDIS_OK ){ /* Handle error */ }
-
- rc = vedis_kv_append_fmt(pStore,
- "msg",-1,"%d:%d:%d",
- 1,12,42); /* msg => 'Hello, Current time is: 10:16:53' */
- /* Store 20 random records.*/
- for(i = 0 ; i < 20 ; ++i ){
- char zKey[12]; /* Random generated key */
- char zData[34]; /* Dummy data */
-
- /* Generate the random key */
- vedis_util_random_string(pStore,zKey,sizeof(zKey));
-
- /* Perform the insertion */
- rc = vedis_kv_store(pStore,zKey,sizeof(zKey),zData,sizeof(zData));
- if( rc != VEDIS_OK ){
- break;
- }
- }
- /* Fetch some data and invoke the supplied callback for each retrieved record* /
- rc = vedis_kv_fetch_callback(pStore,"test",-1,DataConsumerCallback,0);
- if( rc != VEDIS_OK ){/* Can't happen */ }
-
- rc = vedis_kv_fetch_callback(pStore,"msg",-1,DataConsumerCallback,0);
- if( rc != VEDIS_OK ){/* Can't happen */ }
-
- /* Delete a record */
- vedis_kv_delete(pStore,"test",-1);
-
- /* Finally, auto-commit the transaction and close our datastore */
- vedis_close(pStore);
-
Again, the datastore is created on line 5 using a call to vedis_open(). This is often the first Vedis API call that an application makes and is a prerequisite in order to work with the library.
-
Raw data storage via the Key/Value layer is performed on line 9 til line 26 using the various Vedis interfaces such as vedis_kv_store(), vedis_kv_append(), vedis_kv_store_fmt(), vedis_kv_append_fmt(), etc.
-
On line 32 til line 44, we perform an insertion of 20 random records. The random key is obtained by the utility interface: vedis_util_random_string().
- On line 47 til line 50, we fetch our stored data using the powerful vedis_kv_fetch_callback() interface.
-
On line 54, we remove a record from the datastore using vedis_kv_delete() .
-
Finally, the transaction is auto-committed and our datastore is closed on line 57 using the vedis_close() interface.
Other useful Links to start with
Check out the Introduction To The Vedis C/C++ Interface for an introductory overview and roadmap to the dozens of Vedis interface functions. A separate document, The Vedis C/C++ Interface, provides detailed specifications for all of the various C/C++ APIs for Vedis.
Once the reader understands the basic principles of
operation for Vedis, that
document should be
used as a reference guide.
Any questions, visit the Support Page for additional information.
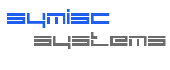